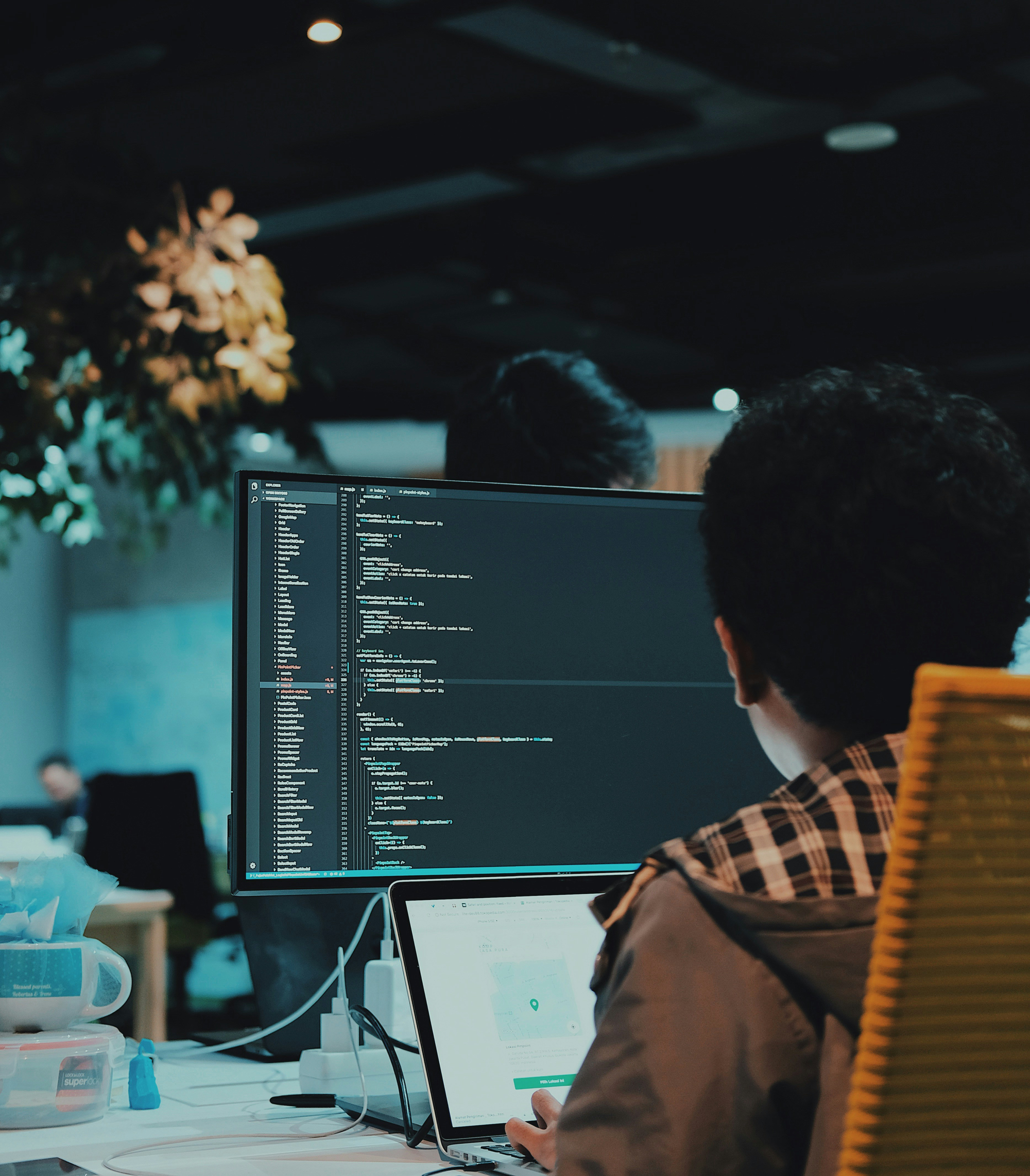
Programming with Python
This course aims to build foundations for programming with python and covers 5 main areas. Each area contains modules, concepts and problem sets.
💡 Prerequisites for the Course
To ensure a smooth learning experience, make sure you meet the following prerequisites:
Hardware:
- A functional laptop or desktop computer
- A reliable internet connection
Software:
- A modern web browser (preferably Google Chrome)
- Zoom for online sessions
Knowledge:
- Basic understanding of calculus
1. Getting Started with Programming
- Creativity, Motivation, and Basics: Understand why programming is important, how it fosters creativity, and the basics of writing programs.
- Understanding Python and Programs: Learn about computer hardware architecture, interpreters vs. compilers, and the building blocks of programs.
- Debugging and Problem Solving: Identify common errors, debug effectively, and build confidence in troubleshooting.
2. Core Concepts
- Variables, Expressions, and Statements: Explore values, types, operators, and how to write meaningful and error-free statements.
- Conditional Execution (if/else): Master boolean logic, alternative executions, and nested/chained conditions for smarter decision-making.
- Loops and Iteration: Learn while/for loops, loop patterns (counting, summing, max/min), and how to handle infinite loops.
3. Working with Data
- Strings: Slicing and Methods: Understand strings as sequences, perform slicing, and use string methods for manipulation and parsing.
- Files: Reading and Writing: Learn file handling basics, how to read/write text files, and search through file contents effectively.
- Lists, Dictionaries, and Tuples: Explore these core data structures, their operations, traversal, and how they interact with other elements like strings.
4. Advanced Programming
- Functions: Writing and Using Them: Use built-in functions, create custom ones, and understand parameters, arguments, and flow of execution.
- Object-Oriented Programming (OOP): Learn to manage larger programs, work with classes/objects, understand inheritance, and apply OOP principles effectively.
- Regular Expressions: Master pattern matching, data extraction, and advanced text parsing techniques.
5. Real-World Applications
- Web Programming and APIs: Learn HTTP basics, retrieve data using urllib, scrape web pages with BeautifulSoup, and work with APIs using XML/JSON.
- Databases and SQL: Understand database concepts, create and query tables, and model relationships with multiple tables.
- Visualizing Data: Build visualizations using geocoded data, explore networks, and analyze real-world datasets.
Know Your Tutor
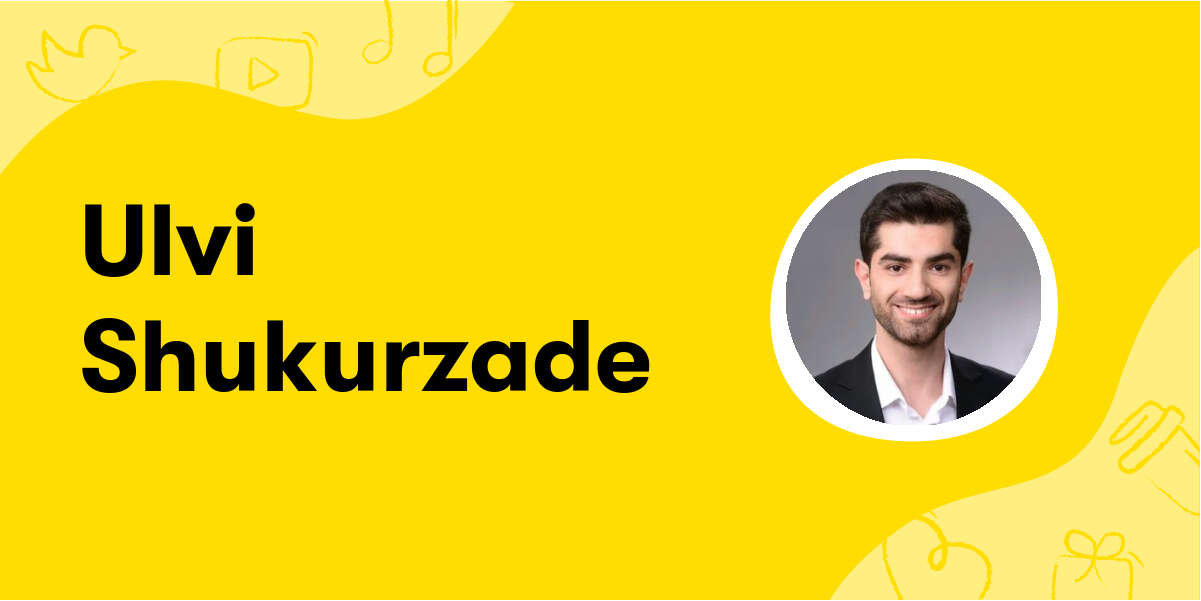
Click to see my CV
Below is a detailed list of the course contents
This course covers a wide range of topics and can be tailored to meet the specific needs of the student. Modules and concepts can be customized, expanded, or omitted based on individual preferences, with the option to focus on areas such as Web Development, Data Visualization, Data Analysis, or Machine Learning, among others.
Each section includes dedicated discussions and hands-on exercise sessions to reinforce learning.
Course Content
1. Why Should You Learn to Write Programs?
- 1.1 Creativity and motivation
- 1.2 Computer hardware architecture
- 1.3 Understanding programming
- 1.4 Words and sentences
- 1.5 Conversing with Python
- 1.6 Terminology: Interpreter and compiler
- 1.7 Writing a program
- 1.8 What is a program?
- 1.9 The building blocks of programs
- 1.10 What could possibly go wrong?
- 1.11 Debugging
- 1.12 The learning journey
- 1.13 Discussion / Revision
- 1.14 Exercises
2. Variables, Expressions, and Statements
- 2.1 Values and types
- 2.2 Variables
- 2.3 Variable names and keywords
- 2.4 Statements
- 2.5 Operators and operands
- 2.6 Expressions
- 2.7 Order of operations
- 2.8 Modulus operator
- 2.9 String operations
- 2.10 Asking the user for input
- 2.11 Comments
- 2.12 Choosing mnemonic variable names
- 2.13 Debugging
- 2.14 Discussion / Revision
- 2.15 Exercises
3. Conditional Execution
- 3.1 Boolean expressions
- 3.2 Logical operators
- 3.3 Conditional execution
- 3.4 Alternative execution
- 3.5 Chained conditionals
- 3.6 Nested conditionals
- 3.7 Catching exceptions using try and except
- 3.8 Short-circuit evaluation of logical expressions
- 3.9 Debugging
- 3.10 Discussion / Revision
- 3.11 Exercises
4. Functions
- 4.1 Function calls
- 4.2 Built-in functions
- 4.3 Type conversion functions
- 4.4 Math functions
- 4.5 Random numbers
- 4.6 Adding new functions
- 4.7 Definitions and uses
- 4.8 Flow of execution
- 4.9 Parameters and arguments
- 4.10 Fruitful functions and void functions
- 4.11 Why functions?
- 4.12 Debugging
- 4.13 Discussion / Revision
- 4.14 Exercises
5. Iteration
- 5.1 Updating variables
- 5.2 The while statement
- 5.3 Infinite loops
- 5.4 Finishing iterations with continue
- 5.5 Definite loops using for
- 5.6 Loop patterns
- 5.6.1 Counting and summing loops
- 5.6.2 Maximum and minimum loops
- 5.7 Debugging
- 5.8 Discussion / Revision
- 5.9 Exercises
6. Strings
- 6.1 A string is a sequence
- 6.2 Getting the length of a string using len
- 6.3 Traversal through a string with a loop
- 6.4 String slices
- 6.5 Strings are immutable
- 6.6 Looping and counting
- 6.7 The in operator
- 6.8 String comparison
- 6.9 String methods
- 6.10 Parsing strings
- 6.11 Formatted String Literals
- 6.12 Debugging
- 6.13 Discussion / Revision
- 6.14 Exercises
7. Files
- 7.1 Persistence
- 7.2 Opening files
- 7.3 Text files and lines
- 7.4 Reading files
- 7.5 Searching through a file
- 7.6 Letting the user choose the file name
- 7.7 Using try, except, and open
- 7.8 Writing files
- 7.9 Debugging
- 7.10 Discussion / Revision
- 7.11 Exercises
8. Lists
- 8.1 A list is a sequence
- 8.2 Lists are mutable
- 8.3 Traversing a list
- 8.4 List operations
- 8.5 List slices
- 8.6 List methods
- 8.7 Deleting elements
- 8.8 Lists and functions
- 8.9 Lists and strings
- 8.10 Parsing lines
- 8.11 Objects and values
- 8.12 Aliasing
- 8.13 List arguments
- 8.14 Debugging
- 8.15 Discussion / Revision
- 8.16 Exercises
9. Dictionaries
- 9.1 Dictionary as a set of counters
- 9.2 Dictionaries and files
- 9.3 Looping and dictionaries
- 9.4 Advanced text parsing
- 9.5 Debugging
- 9.6 Discussion / Revision
- 9.7 Exercises
10. Tuples
- 10.1 Tuples are immutable
- 10.2 Comparing tuples
- 10.3 Tuple assignment
- 10.4 Dictionaries and tuples
- 10.5 Multiple assignment with dictionaries
- 10.6 The most common words
- 10.7 Using tuples as keys in dictionaries
- 10.8 Sequences: strings, lists, and tuples - Oh My!
- 10.9 List comprehension
- 10.10 Debugging
- 10.11 Discussion / Revision
- 10.12 Exercises
11. Regular Expressions
- 11.1 Character matching in regular expressions
- 11.2 Extracting data using regular expressions
- 11.3 Combining searching and extracting
- 11.4 Escape character
- 11.5 Summary
- 11.6 Bonus section for Unix/Linux users
- 11.7 Debugging
- 11.8 Discussion / Revision
- 11.9 Exercises
12. Networked Programs
- 12.1 Hypertext Transfer Protocol - HTTP
- 12.2 The world’s simplest web browser
- 12.3 Retrieving an image over HTTP
- 12.4 Retrieving web pages with urllib
- 12.5 Reading binary files using urllib
- 12.6 Parsing HTML and scraping the web
- 12.7 Parsing HTML using regular expressions
- 12.8 Parsing HTML using BeautifulSoup
- 12.9 Bonus section for Unix/Linux users
- 12.10 Discussion / Revision
- 12.11 Exercises
13. Using Web Services
- 13.1 eXtensible Markup Language - XML
- 13.2 Parsing XML
- 13.3 Looping through nodes
- 13.4 JavaScript Object Notation - JSON
- 13.5 Parsing JSON
- 13.6 Application Programming Interfaces
- 13.7 Security and API usage
- 13.8 Discussion / Revision
14. Object-Oriented Programming (OOP)
- 14.1 Managing larger programs
- 14.2 Getting started
- 14.3 Using objects
- 14.4 Starting with programs
- 14.5 Subdividing a problem
- 14.6 Our first Python object
- 14.7 Classes as types
- 14.8 Object lifecycle
- 14.9 Multiple instances
- 14.10 Inheritance
- 14.11 Summary
- 14.12 Discussion / Revision
15. Using Databases and SQL
- 15.1 What is a database?
- 15.2 Database concepts
- 15.3 Database Browser for SQLite
- 15.4 Creating a database table
- 15.5 Structured Query Language summary
- 15.6 Multiple tables and basic data modeling
- 15.7 Data model diagrams
- 15.8 Automatically creating primary keys
- 15.9 Logical keys for fast lookup
- 15.10 Adding constraints to the data database
- 15.11 Sample multi-table application
- 15.12 Many to many relationships in databases
- 15.13 Modeling data at the many-to-many connection
- 15.14 Summary
- 15.15 Debugging
- 15.16 Discussion / Revision
16. Visualizing Data
- 16.1 Building an OpenStreetMap from geocoded data
- 16.2 Visualizing networks and interconnections
- 16.3 Visualizing mail data